Introduction
As distributed computing grows, event-driven messaging has become critical. Kafka in .NET Core is a powerful solution for handling real-time data ingestion, processing, and delivery. Apache Kafka enables developers to build scalable, decoupled microservices, analytics pipelines, and high-availability event-driven systems.
This guide will cover:
โ Kafkaโs foundational architecture, including its distributed design, partitioning strategy, and high-availability mechanisms.
โ .NET Core integration techniques, demonstrating Kafkaโs role in modern application development.
โ Real-world use cases, illustrating Kafkaโs effectiveness in event-driven applications, telemetry processing, and real-time analytics.
โ A comparative analysis of Kafka versus RabbitMQ and Azure Service Bus, examining scalability, fault tolerance, latency, and deployment considerations.
By the end of this article, readers will have a solid understanding of Kafkaโs role within .NET-based ecosystems, enabling them to make informed architectural decisions for distributed messaging solutions.
Kafkaโs Evolution and Use Cases
Kafka was initially developed by LinkedIn in 2011 to address the demand for high-velocity event ingestion and processing. Traditional messaging paradigms, such as publish-subscribe brokers and queue-based architectures, struggled with handling large-scale, fault-tolerant event streams. Kafka addressed these limitations with a distributed commit log model, ensuring persistent, replayable, and highly scalable message distribution.
๐น Primary Use Cases:
- ๐ Event-driven microservices, enabling asynchronous service communication.
- ๐ Telemetry processing, facilitating real-time monitoring and observability.
- ๐ Log aggregation and centralized event correlation across distributed systems.
- ๐ฌ Scalable queue-based messaging with exactly-once semantics when required.
- ๐ก Streaming analytics, supporting real-time decision-making and machine learning applications.
Kafkaโs Architectural Foundations
Kafka follows a log-based event processing model, distinguishing itself from traditional message queue architectures. The diagram below illustrates Kafkaโs distributed messaging workflow:
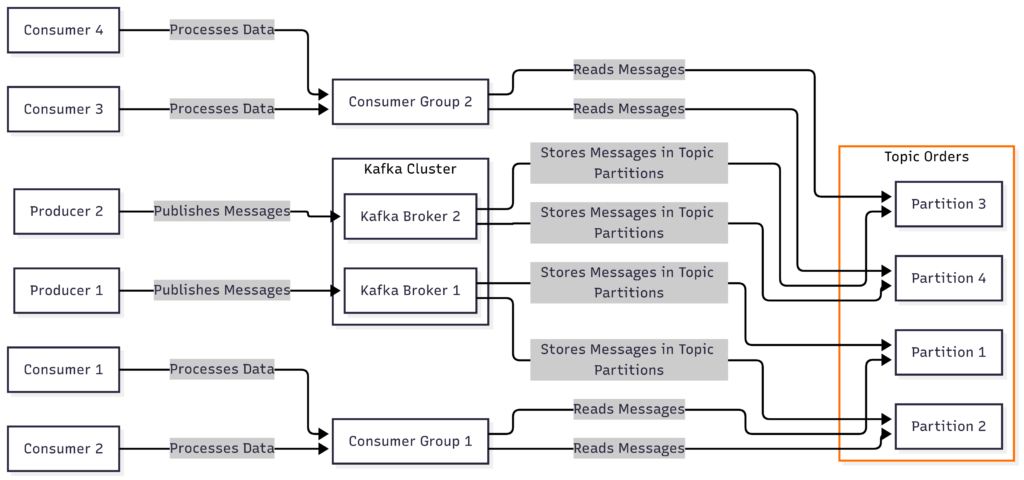
To leverage Kafka in .NET Core for event-driven microservices, developers must understand its partitioning model.
๐น Core Components of Kafka:
- ๐ Producers โ Applications that publish messages to Kafka topics.
- ๐ Topics โ Named channels that store messages, partitioned to enable parallelism.
- ๐ Partitions โ Log segments that provide scalability and redundancy by distributing data across multiple nodes.
- ๐ฅ๏ธ Brokers โ Servers that persist partitioned messages and manage Kafka’s distributed storage.
- ๐ฅ Consumers โ Applications that subscribe to topics and read messages based on assigned offsets.
- ๐ฅ Consumer Groups โ Mechanisms that enable distributed message consumption, ensuring load balancing across multiple consumers.
- ๐ง ZooKeeper (deprecated in favor of KRaft) โ Kafkaโs former metadata manager, handling cluster coordination.
Implementing Kafka in .NET Core
Integrating Kafka into a .NET Core application requires the Confluent.Kafka client library, a widely used package for producing and consuming messages.
๐ฆ Installation
Install Kafkaโs .NET client package using NuGet:
dotnet add package Confluent.Kafka
๐๏ธ Kafka Producer in .NET Core
using Confluent.Kafka;
var config = new ProducerConfig { BootstrapServers = "localhost:9092" };
using var producer = new ProducerBuilder<Null, string>(config).Build();
await producer.ProduceAsync("demo-topic", new Message<Null, string> { Value = "Hello, Kafka!" });
Console.WriteLine("Message successfully dispatched.");
๐น Key Insights:
- ๐ก Establishes a connection with Kafka and writes events to a specified topic.
- ๐ Messages are stored in Kafkaโs partitioned log storage and can be accessed by multiple consumers.
- โก Kafka provides asynchronous message acknowledgment, optimizing throughput.
๐ฅ Kafka Consumer in .NET Core
using Confluent.Kafka;
var config = new ConsumerConfig {
BootstrapServers = "localhost:9092",
GroupId = "demo-group",
AutoOffsetReset = AutoOffsetReset.Earliest
};
using var consumer = new ConsumerBuilder<Null, string>(config).Build();
consumer.Subscribe("demo-topic");
while (true)
{
var message = consumer.Consume();
Console.WriteLine($"Received: {message.Value}");
}
๐น Key Insights:
- ๐ฌ The consumer subscribes to Kafka topics and continuously polls for new messages.
- ๐ Consumer groups ensure partitioned load balancing, allowing horizontal scalability.
- ๐ Offset tracking enables message replayability and fault tolerance.
Kafka vs. RabbitMQ vs. Azure Service Bus: A Comparative Analysis
Kafka, RabbitMQ, and Azure Service Bus cater to different messaging paradigms. The table below compares their architectures and key attributes.
๐น Feature Comparison
Feature | Kafka | RabbitMQ | Azure Service Bus |
---|---|---|---|
โ๏ธ Messaging Model | Event Streaming | Message Queuing | Enterprise Messaging |
โก Throughput | Extremely high | Moderate | Moderate |
๐๏ธ Persistence | Log-based, long retention | Short-lived, per queue | Managed, Azure-hosted |
๐ Message Ordering | Guaranteed per partition | FIFO with additional setup | FIFO supported |
๐ Scalability | Horizontally via partitions | Clustered queues | Cloud-based auto-scaling |
๐ Kafka is Ideal for:
โ High-throughput event streaming and real-time analytics. โ Distributed architectures requiring message persistence. โ Use cases demanding parallel processing and replayability.
๐ RabbitMQ is Best for:
โ Traditional message queuing and task delegation. โ Routing-based messaging architectures using exchange mechanisms. โ Low-latency, single-event processing workflows.
๐ Azure Service Bus is Suited for:
โ Enterprise-grade cloud messaging and workflow automation. โ Secure, reliable messaging for mission-critical applications. โ Seamless integration with Azure services.
FAQs
- Consuming messages from Kafka topic one by one takes too long time. How can I shorten this time? Is reading multiple messages at one time possible?
- How to create a Kafka Topic using Confluent.Kafka .Net Client?
- I’m having trouble to grasp the relationship behind partitions and customer groups.
- In confluent-dotnet-kafka how to pass an object in Message Producer in .Net?
- How to get topics list from Kafka using C#?
- how to write custom serializer for kafka in a .net?
- How to make consume method as non blocking in confluent kafka for dot net?
Conclusion
Kafkaโs dominance in distributed messaging stems from its scalability, persistence, and event replayability, making it a preferred choice for real-time streaming applications, event-driven microservices, and log aggregation frameworks.
Happy Learning! ๐
@devsdaily